- Published on
How to display a GIF from a URL in SwiftUI
- Authors
- Name
- Mick MacCallum
- @0x7fs
Surprisingly, Apple doesn't currently offer any simple APIs to allow you to drop a GIF URL into your app in SwiftUI (or UIKit for that matter). But the good news is, there's a quick workaround for this. We can create a simple SwiftUI wrapper view around a WKWebView, and use it to load the GIF from our URL. In this example, I've disabled the web view's allowsLinkPreview
and allowsBackForwardNavigationGestures
properties to prevent the user from being able to interact with the image, treating it like a normal image view.
import SwiftUI
import WebKit
struct GifImageView: UIViewRepresentable {
private let url: URL
init(url: URL) {
self.url = url
}
func makeUIView(context: Context) -> WKWebView {
let webview = WKWebView()
webview.allowsLinkPreview = false
webview.allowsBackForwardNavigationGestures = false
webview.load(URLRequest(url: url))
return webview
}
func updateUIView(_ uiView: WKWebView, context: Context) {
uiView.reload()
}
}
Then to use this view to display a GIF in your app, just instantiate it and pass a URL. You can even try it with the URL below if you'd like!
GifImageView(
url: URL(string: "https://bleepingswift.com/static/images/articles/gif-image-view/tim.gif")!
)
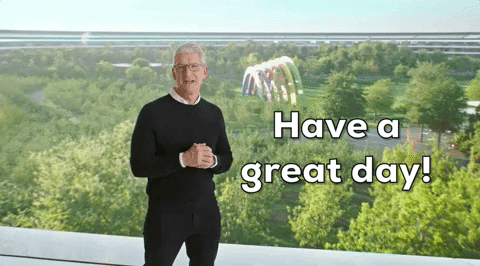